Laravel Pausable Jobs: Selective control over your queue processing
Laravel Pausable Jobs is a package that allows selective pausing and resuming of specific job classes in Laravel queues without stopping entire queue workers.
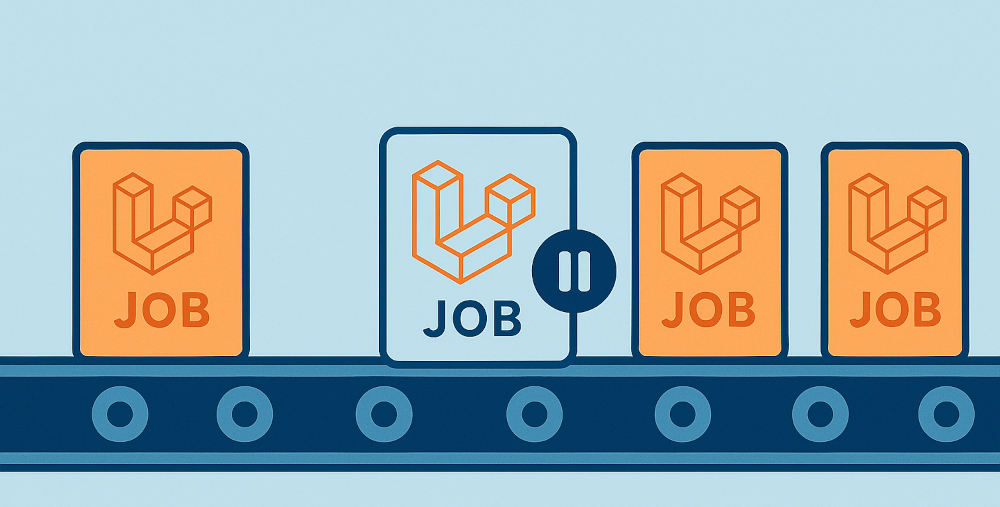
When you’re running a Laravel application with a continuous stream of background jobs, sometimes you need to pause specific job types without bringing your entire queue to a halt. That’s exactly why I built the laravel-pausable-jobs package - to give developers fine-grained control over queue processing.
The Problem: Queue Jobs vs. Database Maintenance
At WhatPulse, we face a constant stream of incoming requests from our desktop application. These requests are transformed into background jobs that process chunks of statistical data. This works brilliantly most of the time, but what happens when you need to perform database maintenance?
Previously, I would stop all queue workers. But this causes data to pile up and creates a backlog that can take hours to clear. What if you could just pause the specific jobs that interact with the database you’re maintaining, while allowing other jobs to continue processing? That’s where Laravel Pausable Jobs comes in.
How It Works
The package is built on a simple premise - add a trait to your job classes, and you’ll gain the ability to pause and resume them individually without affecting other jobs in your queue.
use Smitmartijn\PausableJobs\Concerns\PausableJob;
class ProcessUserStatsJob implements ShouldQueue
{
use PausableJob;
public function handle()
{
// Your job logic here
}
}
Once your job has the PausableJob
trait, you can control its execution with a simple API:
use App\Jobs\ProcessUserStatsJob;
use Smitmartijn\PausableJobs\Facades\JobPause;
// Need to do database maintenance? Pause only the jobs that would be affected
JobPause::pauseJobClass(ProcessUserStatsJob::class);
// When maintenance is complete, resume processing
JobPause::resumeJobClass(ProcessUserStatsJob::class);
// You can also check the current status
if (JobPause::isPaused(ProcessUserStatsJob::class)) {
// Job is paused
}
Real-World Usage at WhatPulse
At WhatPulse, this package has become an essential tool for our operations. When we need to perform maintenance on specific database tables or run heavy migrations, we simply pause the relevant jobs. This means the application continues to accept incoming data from users, and only the processing of that specific data type is temporarily halted - to be resumed once the maintenance is done.
Another use case is when we need to pause jobs that interact with external APIs. By selectively pausing these jobs, we can prevent unnecessary API calls during maintenance windows or when the API is down.
Getting Started
Installing the package is straightforward:
composer require smitmartijn/laravel-pausable-jobs
php artisan vendor:publish --tag=pausable-jobs-config
The published configuration file allows you to customize behavior like retry times, storage driver (Redis or Cache), and logging options.
Bonus: Filament Admin Widget
For those using Filament, the package includes an example widget that provides a visual overview of all pausable jobs. This makes it even easier to monitor and control the state of your queue processing directly from your admin panel.
Compatibility and Requirements
The package works with:
- PHP 8.1+
- Laravel 10+
- Multiple queue workers
- Laravel Horizon
Final Thoughts
Building Laravel Pausable Jobs came from a real need at WhatPulse, but it’s applicable to any Laravel application with a busy queue system. Being able to pause specific job classes has saved us countless hours of maintenance downtime and prevented the backlog issues that used to plague our queue processing.
If you’ve found this package useful or have ideas for improvements, feel free to post an issue on the Github repository, or give me a shout on Bluesky. 😊